What is a Validation URL?
When you add a Validation URL to your sheet, Orca Scan sends the data entered into the Orca mobile app to your URL to be validated before it is saved.
What can I do with the Validation URL?
You can use the Validation URL to:
- Inspect data before it is saved
- Reject data with an error message
- Modify data before it is saved
- Send a notification to the mobile app
How does the Validation URL work?
Let’s say a user scans a QR code containing an email address, and enters comments into a notes
field. A HTTP POST will be sent to your URL containing:
{
"___orca_sheet_name": "Vehicle Checks",
"___orca_user_email": "hidden@requires.https",
"barcode": "hello@orcascan.com",
"notes": "Something important!"
}
Along with the following HTTP Headers:
Parameter | Description |
---|---|
orca-sheet-id |
Unique ID of the Orca Scan sheet |
orca-sheet-name |
The name of the sheet making the request |
orca-user-email |
Email of user scanning the barcode (requires HTTPS) |
orca-timestamp |
UNIX epoch time of the request |
orca-secret |
Sheet assigned secret (check this to verify request) |
orca-request-type |
validation |
Allowing you to inspect the data and decided if you should accept or reject.
How do I reject invalid data?
Return a HTTP status code 400 to reject data.
How do I show an error dialog in the app?
Return a HTTP status code 400 along with an ___orca_message
with display set to dialog and type set to error, for example:
{
"___orca_message": {
"display": "dialog",
"type": "error",
"title": "Validation Error",
"message": "Please provide more information"
}
}
A dialog will appear and the user will be unable to proceed until resolved:
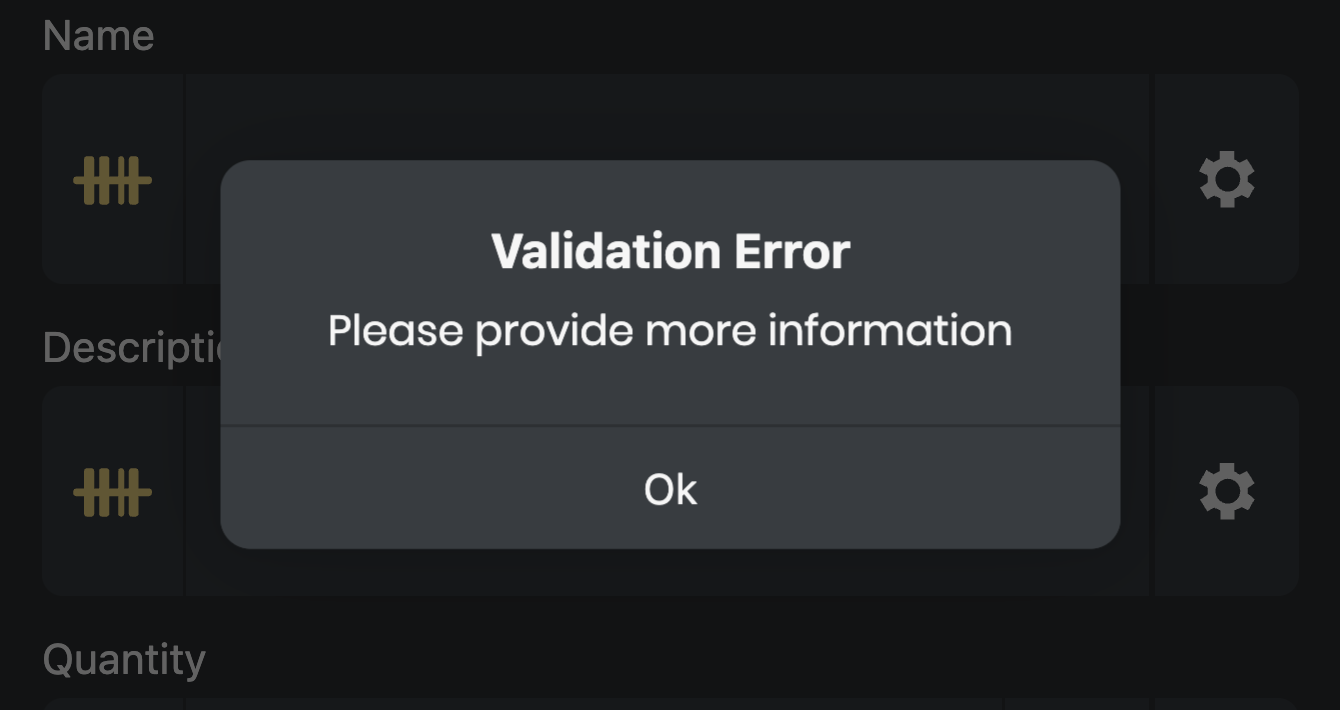
How do I show a notification in the app?
Return an ___orca_message
with display set to notification, for example:
{
"___orca_message": {
"display": "notification",
"type": "success",
"message": "Please scan next item"
}
}
A notification will appear briefly at the top of the app:
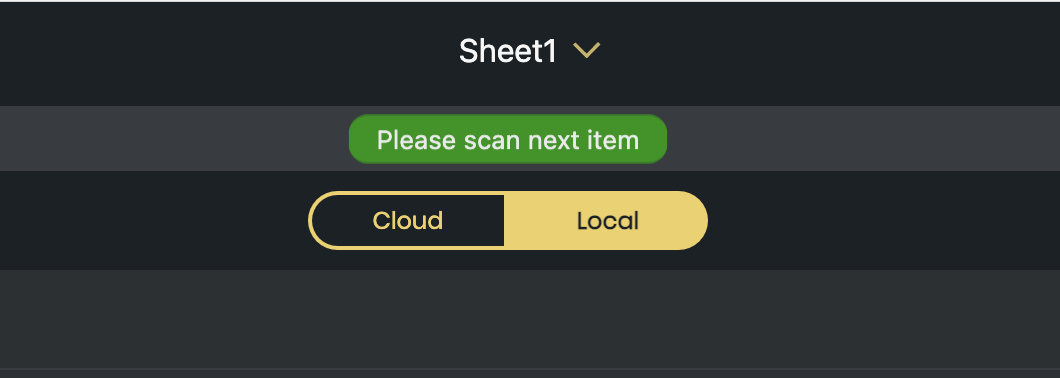
There are three types of notifications to choose from:
Type | Appearance |
---|---|
error |
A red notification appears at the top of the app. |
warning |
A yellow notification appears at the top of the app. |
success |
A green notification appears at the top of the app. |
Can the Validation URL modify the data?
Yes. Simply return a HTTP status code 200 along with the modified data as a JSON object. The Orca barcode app will apply these changes, and, if no ___orca_message
is present, allow the user to continue to the next task:
{
"barcode": "hello@orcascan.com",
"notes": "Not that important :)"
}
How do I create a Validation URL?
You need to create an endpoint that accepts a HTTP POST. Once configured you will be able to accept or reject data depending on your code, or use it to guide the user into what action to perform next using a notification
. Here are a few examples:
const app = express();
app.use(express.json());
app.post('/', function(request, response){
data = request.body;
// dubug purpose: show in console raw data received
console.log("Request received: \n"+JSON.stringify(data, null, 2));
// orca system fields start with ___
// access the value of fields using field name
// example: data.Name, data.Barcode, data.Location
const name = data.Name
// validation example
if (name.length > 20) {
// return error message to show user
response.json({
"title": "Invalid Name",
"message": "Name cannot contain more than 20 characters",
}).send();
return;
}
// return HTTP Status 204 (no content)
response.status(204).send();
});
app.listen(3000, () => console.log('Example app is listening on port 3000.'));
[ApiController]
[Route("/")]
public class OrcaValidationDotNet : ControllerBase
{
[HttpPost]
[Consumes("application/json")]
public async Task<ActionResult> validationReceiver()
{
using (StreamReader reader = new StreamReader(Request.Body, Encoding.UTF8))
{
// get the raw JSON string
string json = await reader.ReadToEndAsync();
// convert into .net object
dynamic data = JObject.Parse(json);
// orca system fields start with ___
// access the value of fields using field name
// example: data.Name, data.Barcode, data.Location
string name = (data.Name != null) ? (string) data.Name : "";
// validation example
if (name.Length > 20) {
// return error message to show user
return Ok(new {
title = "Name is too long",
message = "Name cannot contain more than 20 characters"
});
}
}
// return HTTP Status 204 (no content)
return NoContent();
}
}
func validationHandler(w http.ResponseWriter, r *http.Request) {
// Read body
body, err := ioutil.ReadAll(r.Body)
defer r.Body.Close()
if err != nil {
fmt.Println(err)
http.Error(w, err.Error(), 500)
return
}
// Parse JSON data
var barcode OrcaBarcode
jsonErr := json.Unmarshal([]byte(body), &barcode)
if jsonErr != nil {
fmt.Println(jsonErr)
http.Error(w, jsonErr.Error(), 500)
return
}
// dubug purpose: show in console raw data received
fmt.Println(barcode)
// orca system fields start with ___
// access the value of fields using field name
// example: data.Name, data.Barcode, data.Location
name := barcode.Name
// validation example
if (len(name) > 20) {
// return error message to show user
w.Write([]byte(`{
"title": "Invalid Name",
"message": "Name must be less than 20 characters"}
`))
return
}
// return HTTP Status 204 (no content)
w.WriteHeader(204)
}
@app.route('/', methods=['POST'])
def orca_validation():
if request.method == 'POST':
data = request.get_json()
# dubug purpose: show in console raw data received
print("Request received: \n"+json.dumps(data, sort_keys=True, indent=4))
# orca system fields start with ___
# access the value of fields using field name
# example: data["Barcode"], data["Location"]
name = data["Name"]
# validation example
if(len(name) > 20):
# return error message to show user
return json.dumps({
"title": "Invalid Name",
"message": "Name cannot contain more than 20 characters",
})
# return HTTP Status 204 (no content)
return '', 204
if (preg_match('/$/', $_SERVER["REQUEST_URI"])) {
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$data = json_decode(file_get_contents('php://input'), true);
// orca system fields start with ___
// access the value of fields using field name
// example: data.Name, data.Barcode, data.Location
$name = $data["Name"];
// validation example
if (strlen($name) < 20) {
// return error message to show user
echo json_encode(array(
"title" => "Invalid Name",
"message" => "Name cannot contain more than 20 characters"
));
exit;
}
// return HTTP Status 204 (No Content)
http_response_code(204);
exit;
}
}
@RequestMapping(
value = "/",
method = RequestMethod.POST)
String index(@RequestBody Map<String, Object> data) throws Exception {
// dubug purpose: show in console raw data received
System.out.println(data);
// orca system fields start with ___
// access the value of fields using field name
// example: data.get("Barcode"), data.get("Location")
String name = data.get("Name").toString();
// validation example
if (name.length() > 20) {
// return error message to show user
JSONObject json = new JSONObject();
json.put("title", "Invalid Name");
json.put("message", "Name cannot contain more than 20 characters");
return json.toJSONString();
}
// return HTTP Status 204 (no content)
return "";
}
Full working examples for each language are available on GitHub.
How do I add a Validation URL to my sheet?
1. Edit Sheet Integrations
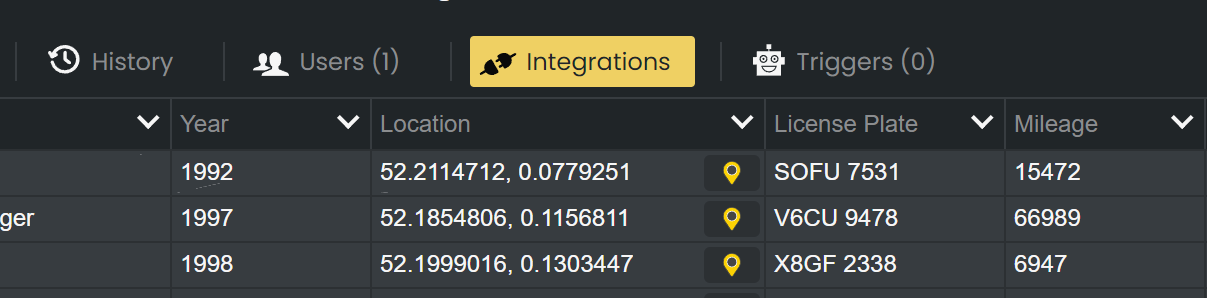
A Validation URL can only be set on sheets created at cloud.orcascan.com. To add a Validation URL, select the sheet you would like to validate, then open the Integration Settings.
2. Add your Validation URL
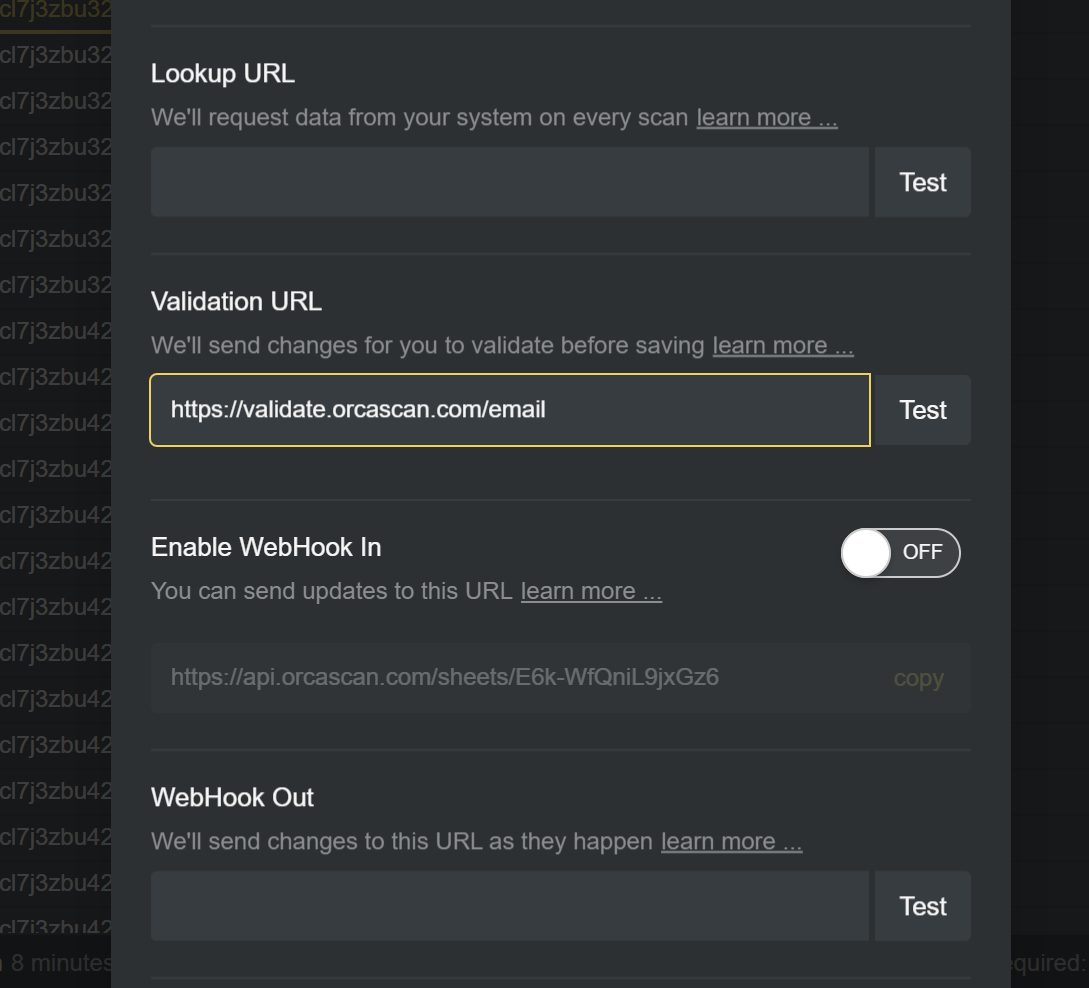
Now enter the Validation URL you would like Orca Scan to call as items are updated. Please note: this URL must be publicly accessible.
Security
You can provide a secret that will be sent as an HTTP header with every request; allowing you to confirm the request is from Orca Scan.
3. Test your Validation URL
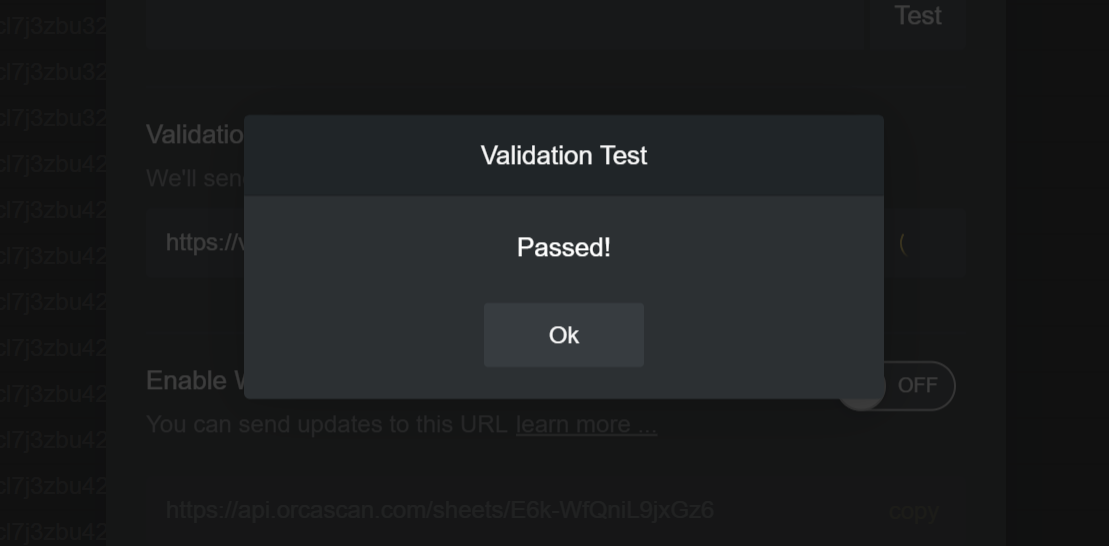
You can now test the Validation URL by clicking the Test button. This will HTTP POST a JSON object to your server containing randomly generated data and display the result.
To test an error, simply have your endpoint return a JSON object containing a title and message and hit test again.
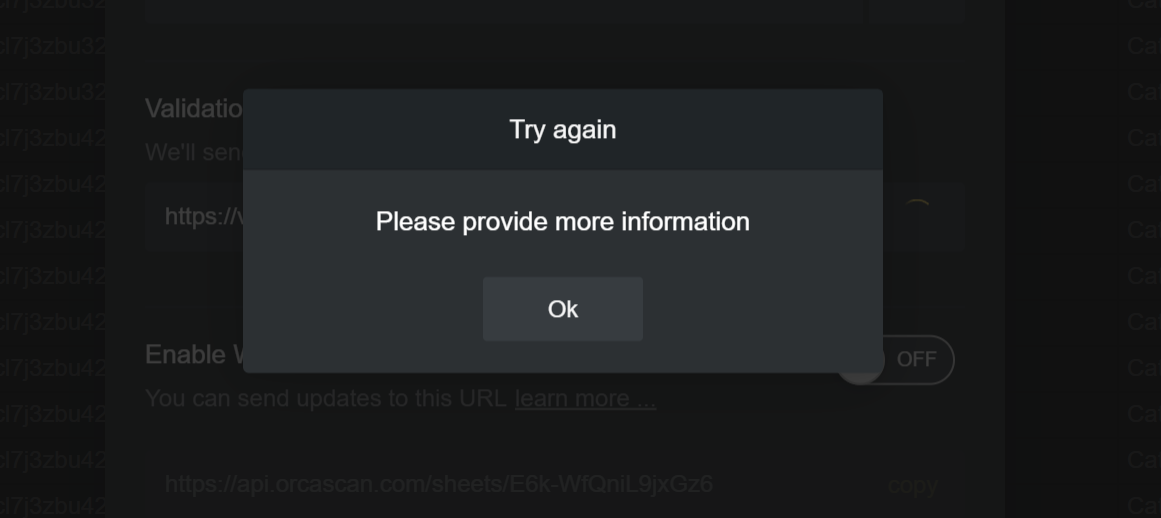
4. Save the changes
Finally, save the changes and you’re done. You can now select that sheet in the Orca Scan mobile app and test it.
Validation URL questions?
We’re happy to help you get up and running, chat with us live or drop us an email.